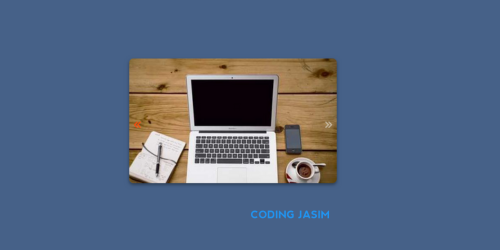
An image slider is a type of graphical user interface (GUI) that displays images or slides in a single location. The slider usually contains a set of controls that enable users to navigate through the slides, pause or play the sideshow, and adjust the timing and speed of the transitions. Some image sliders are also allow users to add captions, descriptions, or links to each slide, giving them more context and interactivity.
Also this feature is commonly found on most of the websites showcasing a significant amount of visual material such as photography, online shopping, and news platforms. Image sliders are often referred to as image carousals or image galleries.
There are many types of image sliders, each with its own features and functionality.
Now we learn about Basic Image Slider – This is a simple image slider that displays a set of images in a sequential order. It usually has double right & left vector buttons to move back and forth between slides
This below short demo of Image Slider for your reference.
Lets start with coding part, as per demo first create a folder and you can name this folder whatever you want, and inside this folder first create an file index.html to structure the web page as per our requirements.
<!DOCTYPE html>
<!--Coding by Coding Jasim - www.codingjasimweb.com -->
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Image Slider | Coding Jasim</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.2.1/css/all.min.css" integrity="sha512-MV7K8+y+gLIBoVD59lQIYicR65iaqukzvf/nwasF0nqhPay5w/9lJmVM2hMDcnK1OnMGCdVK+iQrJ7lzPJQd1w==" crossorigin="anonymous" referrerpolicy="no-referrer" />
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="slider-container">
<div class="image-container">
<!--images selection from Lorem Picsum picsum.photos then select specific image and then copy the any one image link as you want-->
<img src="https://picsum.photos/id/8/500/350" alt="image">
<img src="https://picsum.photos/id/77/500/350" alt="image">
<img src="https://picsum.photos/id/13/500/350" alt="image">
<img src="https://picsum.photos/id/2/500/350" alt="image">
<img src="https://picsum.photos/id/25/500/350" alt="image">
</div>
<!--getting buttons from font awesome-->
<i class="fa-solid fa-angles-left btn prev"></i>
<i class="fa-solid fa-angles-right btn next"></i>
</div>
<script src="index.js"></script>
</body>
</html>
body{
margin: 0; display: flex;justify-content: center;height: 100vh;
align-items: center; background-color: rgba(70, 97, 136);
}
.slider-container{
position: relative;/*2) now .btn icon found inside top left corner of this slider-container*/
width: 500px; height: 300px; overflow: hidden; border-radius: 10px;
box-shadow: 0 4px 8px rgba(0,0,0, 0.4);
}
.image-container{
display: flex; transition:transform 0.5s ease-in-out ;
}
.btn{
position: absolute;/*1) to bring 'btn' inside the container, so it is relative to parent i.e slider-container*/
top: 50%;/*3) now buttons are visible at center*/
color: white; font-size: 20px; opacity: 0.5; cursor: pointer;
}
.btn:hover{
opacity: 1; color: orangered;
}
.btn.prev{
left: 10px;
}
.btn.next{
right: 10px;
}
Now inside the same folder lets create an file style.css, to decorate and design the layout of the page which will achieves our requirements.
Finally, create an file called index.js on the same folder. In which you add some interactive behavior on the page by using methods, functions, etc. In this image slider case we use methods line querySelect(), addEventListener() with some functions clearTimeout() and setTimeout()
const nextEl = document.querySelector(".next");
const prevEl = document.querySelector(".prev");
const imagecontainerEl = document.querySelector(".image-container");
//to know total image count then the below code is
const imgCountEl = document.querySelectorAll("img");
let timeOut; //crucial point (cp)
let currentImg = 1;
nextEl.addEventListener("click", ()=>{
currentImg++;
clearTimeout(timeOut);//cp
updateImg();
});
prevEl.addEventListener("click", ()=>{
currentImg--;
clearTimeout(timeOut);//cp
updateImg();
});
function updateImg(){
if (currentImg > imgCountEl.length){
currentImg = 1;
}else if(currentImg < 1){
currentImg = imgCountEl.length;
}
//to view next image after clicking next btn then code is
imagecontainerEl.style.transform = `translateX(-${(currentImg - 1) * 500}px)`;
timeOut = setTimeout(() => {
currentImg++;
updateImg();
},4000);
}
updateImg();