Discover how to develop a personalized slug generator using HTML, CSS, and JavaScript. Our comprehensive guide will walk you through the process of constructing and testing your own slug generator.
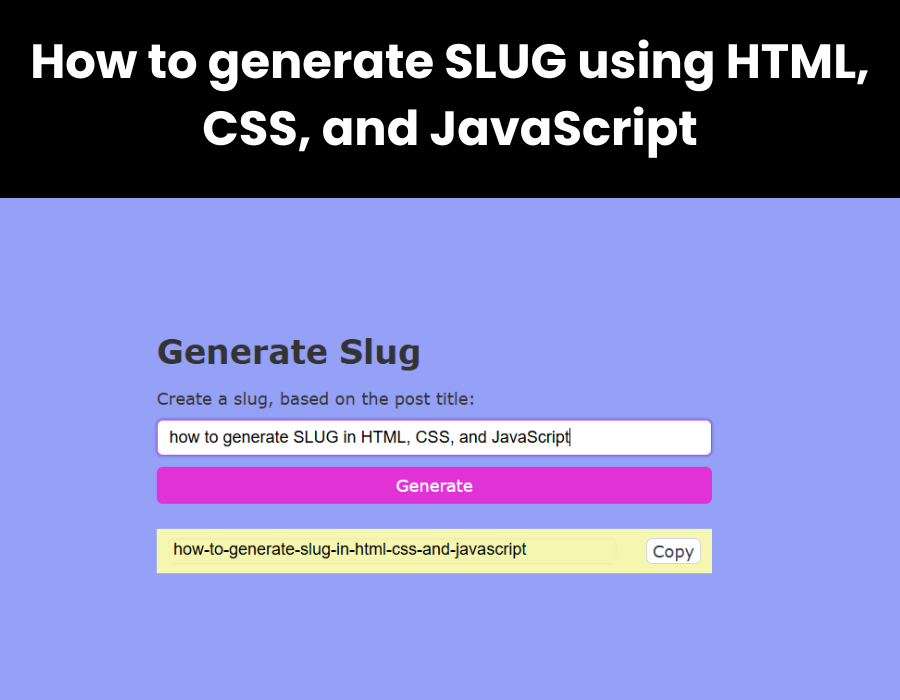
<!DOCTYPE html>
<html lang="en">
<head>
<title>Custom Slug Generator</title>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width" />
<link rel="stylesheet" href="style.css" />
</head>
<body>
<header>
<h1>Generate Slug</h1>
</header>
<main>
<form id="generate">
<label for="title">Create a slug, based on the post title:</label>
<input type="text" name="title" id="title" placeholder="Slug is needed for this title" onfocus="this.value=''">
<button class="primary-button" type="submit">Generate</button>
</form>
<div id="result">
<input type="text" ></input>
<button class="default-button" id="copy" type="button">Copy</button>
</div>
</main>
<script src="script.js"></script>
</body>
</html>
html {
--clr-white: #ffffff;
--clr-primary: hsl(304, 74%, 54%);
}
*, *::before, *::after {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family:Verdana, Geneva, Tahoma, sans-serif;
font-size: 18px;
background-color: #94a1f6;
color: #333333;
padding: 2rem;
min-height: 100vh;
display: flex;
flex-direction: column;
justify-content: center;
align-items: stretch;
}
body > * + * {
margin-top: 1em;
}
header, main {
max-width: 600px;
width: 100%;
margin-left: auto;
margin-right: auto;
}
#generate {
--paddingX: calc(.75em - 1px);
--paddingY: calc(.5em - 1px);
--borderR: 0.375em;
display: flex;
flex-direction: column;
align-items: stretch;
gap: 0.75rem;
}
input[type="text"] {
font-size: 1em;
background-color: var(--clr-white);
border: 1px solid #dbdbdb;
border-radius: var(--borderR);
padding: var(--paddingY) var(--paddingX);
box-shadow: inset 0 0.0625em 0.125em rgb(10 10 10 / 5%);
}
input[type="text"]:focus {
outline: none;
border-color: var(--clr-primary);
box-shadow: 0 0 0 0.125em rgb(72 95 199 / 25%);
}
button {
text-align: center;
font: inherit;
color: var(--color);
background: var(--bgColor);
border: 1px solid var(--borderColor);
border-radius: var(--borderR);
padding: var(--paddingY) var(--paddingX);
margin: 0;
display: block;
cursor: pointer;
}
button:focus {
outline-offset: 2px;
}
.primary-button {
--color: var(--clr-white);
--bgColor: var(--clr-primary);
--borderColor: var(--clr-primary);
}
.default-button {
--color: hsl(217deg 23% 27%);
--bgColor: var(--clr-white);
--borderColor: hsl(216deg 16% 84%);
}
#result {
visibility: hidden;
--paddingX: 6px;
--paddingY: 2px;
--borderR: 0.375em;
background-color: hsl(62, 82%, 83%);
color: #da1039;
padding: calc(var(--paddingY) * 5) calc(var(--paddingX) * 2);
margin-top: 1.5em;
display: flex;
align-items: flex-start;
gap: 2rem;
}
#result input {
font-size: 1em;
background: transparent;
border: none;
height: auto;
resize: none;
display: block;
flex: 1;
}
const create = document.getElementById("generate");
const output = document.querySelector("#result input");
const copy = document.getElementById("copy");
const showDiv = document.getElementById("result");
create.addEventListener("submit", function (e) {
e.preventDefault();
const title = e.target.title.value;
const slug = title.toLowerCase().split(" ").join("-").replace(/[:.,?!]/gi, "");
if (output) {
output.value = slug;
e.target.title.value = "";
showDiv.style.visibility = "visible";
} else {
console.error("Sth wrong...");
}
});
function copy2clipboard() {
let custom_code = output.select();
document.execCommand('copy');
}
copy.addEventListener("click", copy2clipboard);