Discover the steps to craft a secure login form featuring a captcha, all with HTML, CSS, and JavaScript. Elevate the level of user authentication and security on your site.
In the era of the internet, the importance of online safety is at an all-time high for both website creators and visitors. A key element in safeguarding user information and ensuring a secure online journey is the establishment of a strong login mechanism. A crucial part of this mechanism is a login form that incorporates a Captcha.
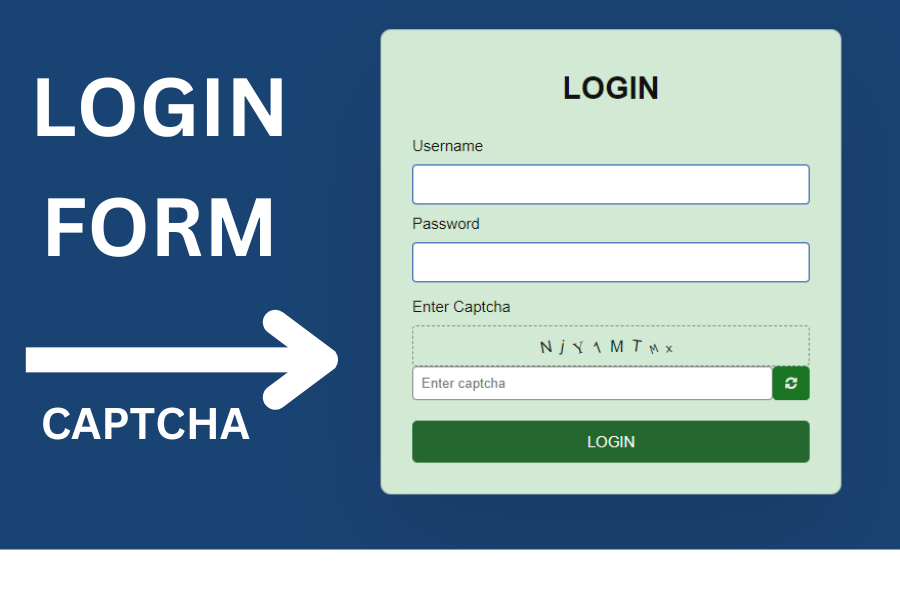
This article is designed to walk you through the journey of developing a secure and user-friendly captcha login form, harnessing the capabilities of HTML, CSS, and JavaScript. By adhering to these instructions, you can greatly bolster the security of your site, shield user accounts from unauthorized entry, and offer a smooth and intuitive login process. Let’s begin our exploration into crafting a login form that achieves the ideal equilibrium between user satisfaction and security.
HTML Code :
To build a successful login form, start by setting up the HTML framework. Follow these steps:
- Start with a <form> to hold the login features.
- Include <label> and <input> tags for the username and passwords.
- Place a submit <button> for user’s login purpose.
- Add identifiers and placeholders for ease of use.
- Organize your HTML code in a neat and systematic way.
In addition, the below script tag connects to an outside JavaScript library known as “sweetalert”. It’s utilize for generating pop-up alerts or message on the website.
<script src=”https://unpkg.com/sweetalert/dist/sweetalert.min.js”></script>
<!DOCTYPE html>
<html lang="en">
<head>
<title>Login Form with Captcha | Coding Jasim</title>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width" />
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css">
<link rel="stylesheet" href="styles.css" />
</head>
<body>
<div class="login-form">
<div class="form-title">
LOGIN
</div>
<div class="form-input">
<label for="username">Username</label>
<input type="text" id="username">
</div>
<div class="form-input">
<label for="password">Password</label>
<input type="password" id="password">
</div>
<div class="captcha">
<label for="captcha-input">Enter Captcha</label>
<div class="preview"></div>
<div class="captcha-form">
<input type="text" id="captcha-form" placeholder="Enter captcha">
<button class="captcha-refresh">
<i class="fa fa-refresh"></i>
</button>
</div>
</div>
<div class="form-input">
<button id="login-btn">Login</button>
</div>
</div>
<script src="https://unpkg.com/sweetalert/dist/sweetalert.min.js"></script>
<script src="script.js"></script>
</body>
</html>
CSS Code :
Apply CSS techniques to enhance the appearance and ease of use of your login page.
- Incorporate CSS techniques on components such as form, labels, and input areas.
- Employ responsive design to guarantee it works well on different gadgets.
- Think about color palettes, fonts, and the arrangement of content for a refined appearance.
* {
margin:0px;
padding:0px;
box-sizing:border-box;
}
body {
font-family:sans-serif;
background:#194372;
}
.login-form {
position:absolute;
top:50%;
left:50%;
transform:translate(-50%,-50%);
width:90%;
max-width:450px;
background:#d2ead3;
padding:20px 30px;
box-shadow: rgba(17, 12, 46, 0.15) 0px 48px 100px 0px;
border: 1px solid rgba(17, 12, 46, 0.15);
border-radius: 10px;
}
.login-form .form-title {
text-align:center;
font-size:30px;
font-weight:600;
margin:20px 0px 30px;
color:#111;
}
.login-form .form-input {
margin:10px 0px;
}
.login-form .form-input label,
.login-form .captcha label {
display:block;
font-size:15px;
color:#111;
margin-bottom:10px;
}
.login-form .form-input input {
width:100%;
padding:10px;
outline: none;
border-radius: 4px;
/*border:1px solid #888;*/
border:1px solid #1e4bb2;
font-size:15px;
}
.login-form .captcha {
margin:15px 0px;
}
.login-form .captcha .preview {
color:#131212;
width:100%;
text-align:center;
height:40px;
line-height:40px;
letter-spacing:8px;
border:1px dashed #888;
border-radius: 4px;
font-family:"monospace";
}
.login-form .captcha .preview span {
display:inline-block;
user-select:none;
}
.login-form .captcha .captcha-form {
display:flex;
}
.login-form .captcha .captcha-form input {
width:100%;
outline: none;
padding:8px;
border-radius: 4px;
border:1px solid #888;
}
.login-form .captcha .captcha-form .captcha-refresh {
width:40px;
border:none;
outline:none;
background:#197522;
border-radius: 4px;
color:#eee;
cursor:pointer;
}
#login-btn {
margin-top:5px;
width:100%;
padding:12px;
border:none;
outline:none;
font-size:15px;
text-transform:uppercas;
background:#246830;
border-radius: 5px;
color:#fff;
transition: .3s;
cursor:pointer;
}
#login-btn:hover{
opacity: 0.7;
}
JavaScript Code :
To verify user input and deliver immediate feedback, utilize JavaScript:
- Create JavaScript functions to check the username and password fields.
- Verify that it is formatted correctly, is the right length and contains no malicious code.
- Show success indicators or error warnings instantly.
Allow me to dissect the code in detail:
a. A array named fonts is created, which holds four different font styles:”cursive,” “sans-serif,” “serif,” and “monospace.”
b. A variable named captchaVal is declared and given an initial value of an empty string, which holds the current captcha value.
c. The program outlines three functions:
1. produceCaptcah: This function creates a fresh captcha value. It achieves this by executing the following actions:
- It produces a random integer between 0 and 1 and scales it by up by 1,000,000,000.
- It transforms this number into base64 with btoa.
- It shortens the base64 string to a range of 5 to 10 characters by selecting a random length.
- The outcome is saved in the captchaVal variable.
2. corCaptcha: This method generates the captcha image and inserts it into the HTML document. It produces and HTML string that encompasses every character of the captcha. For each character:
- It picks a random angle for rotation between -20 and +10 degrees.
- It chooses a random font from the list of fonts.
- It forms an HTML <span> element with the character, the selected rotation angle, and font.
- These HTML elements are joined together into one string and assigned as the content of a div with the class “preview” inside a div with the class “captcha” within a form.
3. initiateCaptcha: This method sets up the captcha system by attaching an event listener to a “captcha-refresh” button. Upon clicking this button, it refreshes the captcha and updates the visual display by invoking producCaptcha and corCaptcha. Additionally, it initializes and sets the captcha upon the page’s initial load.
e. The code attaches an event listener to the “login” button. Upon button click, it verifies the input matches the current captcha value. If the input is match, it shows a success message through a function named swal. If the input does not match, it shows an “Invalid captcha” message by using the same swal function.
(function(){
const fonts = ["cursive","sans-serif","serif","monospace"];
let captchaVal = "";
function produceCaptcha(){
let value = btoa(Math.random()*1000000000);
value = value.substr(0,5+Math.random()*5);
captchaVal = value;
}
function corCaptcha(){
let html = captchaVal.split("").map((char)=>{
const circulate = -20 + Math.trunc(Math.random()*30);
const font = Math.trunc(Math.random()*fonts.length);
return `<span
style="
transform:circulate(${circulate}deg);
font-family:${fonts[font]}
"
>${char}</span>`;
}).join("");
document.querySelector(".login-form .captcha .preview").innerHTML = html;
}
function initiateCaptcha(){
document.querySelector(".login-form .captcha .captcha-refresh").addEventListener("click",function(){
produceCaptcha();
corCaptcha();
});
produceCaptcha();
corCaptcha();
}
initiateCaptcha();
document.querySelector(".login-form #login-btn").addEventListener("click",function(){
let inputCaptchaValue = document.querySelector(".login-form .captcha input").value;
if(inputCaptchaValue === captchaVal){
swal("", "Logging In!", "success");
} else {
swal("Invalid captcha");
}
});
})();
Conclusion :
To sum up, developing a strong and easy-to-use login page with a Captcha feature using HTML, CSS, and JavaScript is a vital step in improving the safety of your website. As online threats grow more sophisticated, it’s more critical than ever to protect user information and accounts.
By following the instructions provided in this manual, you can find the ideal equilibrium between safety and ease of use. This approach not only shields your visitors from harmful individuals but also ensures a seamless and hassle-free login experience.
Keep in mind, a well-crafted login page showcases your dedication to the security and satisfaction of your users. So don’t hesitate to apply these methods to strengthen your website’s login feature and ensure the protection of your users.