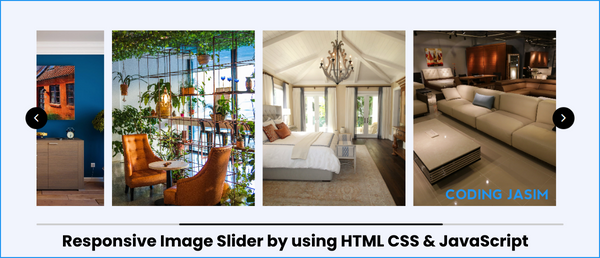
A responsive image slider is a dynamic web element that showcases a sequence of images in visually appealing manner, adapting seamlessly to different screen sizes and devices. This tool is commonly employed in websites to enhance user experience and engage visitors with a visually compelling display.
By using HTML, CSS, and JavaScript developers can learn how this image sliders work and the code required to build it.
In this image slider, there are two buttons for sliding/navigating through the images: one for viewing the previous image and another for displaying the next image in sequence upon click. Below the images, there is a horizontal scroll bar that serve as an indicator between the images, allowing users to slide through them by dragging over it. This kind of image carousal is compatible with all major web browsers, including Chrome, Firefox, and Edge, among others.
Step-by-step guide to demonstrate this image slider by using HTML and JS
- Let’s set up a directory with a name of your choice and then generate the essentials files within it.
- For the primary HTML code, create a file as index.html
- For the CSS styling, create a file Style.css
- For JavaScript functionality, create a file script.js
- Then finally, create a folder named Images and placed all the required images that you have downloaded inside it.
HTML Code
Now lets start with code to your index.html file as follows, which is main structural part of your page it includes all HTML tags such as div, button, img, etc.,
<!DOCTYPE html>
<!-- Coding By Coding Jasim - www.codingjasimweb.com -->
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Image Slider | Coding Jasim</title>
<!-- Google Fonts Link For Icons -->
<link
rel="stylesheet"
href="https://fonts.googleapis.com/css2?family=Material+Symbols+Rounded:opsz,wght,FILL,GRAD@48,400,0,0"
/>
<link rel="stylesheet" href="style.css" />
<script src="script.js" defer></script>
</head>
<body>
<div class="container">
<div class="slider-wrapper">
<button id="prev-slide" class="slide-button material-symbols-rounded">
chevron_left
</button>
<ul class="image-list">
<img class="image-item" src="images/image1.jpg" alt="img-1" />
<img class="image-item" src="images/image2.jpg" alt="img-2" />
<img class="image-item" src="images/image3.jpg" alt="img-3" />
<img class="image-item" src="images/image4.jpg" alt="img-4" />
<img class="image-item" src="images/image5.jpg" alt="img-5" />
<img class="image-item" src="images/image6.jpg" alt="img-6" />
<img class="image-item" src="images/image7.jpg" alt="img-7" />
<img class="image-item" src="images/image8.jpg" alt="img-8" />
<img class="image-item" src="images/image9.jpg" alt="img-9" />
<img class="image-item" src="images/image10.jpg" alt="img-10" />
</ul>
<button id="next-slide" class="slide-button material-symbols-rounded">
chevron_right
</button>
</div>
<div class="slider-scrollbar">
<div class="scrollbar-track">
<div class="scrollbar-thumb"></div>
</div>
</div>
</div>
</body>
</html>
Next, incorporate the following CSS codes into your style.css file to enhance the aesthetic of your image slider. Feel free to experiment around with various CSS properties such as colors, fonts, and backgrounds to add a unique flair to your slider. Once you open the web page in your browser, you’ll be able to view your image slider complete with a scroll bar and an arrow button.
* {
margin: 0;padding: 0;box-sizing: border-box;
}
body {
display: flex;align-items: center;justify-content: center;
min-height: 100vh;background: #f1f4fd;
}
.container { max-width: 1200px; width: 95%;
}
.slider-wrapper {
position: relative;
}
.slider-wrapper .slide-button {
position: absolute; top: 50%; outline: none;border: none;
height: 50px; width: 50px; z-index: 5; color: #fff;
display: flex; cursor: pointer; font-size: 2.2rem; background: #000;
align-items: center;justify-content: center; border-radius: 50%;
transform: translateY(-50%);
}
.slider-wrapper .slide-button:hover {
background: #404040;
}
.slider-wrapper .slide-button#prev-slide {
left: -25px; display: none;
}
.slider-wrapper .slide-button#next-slide {
right: -25px;
}
.slider-wrapper .image-list {
display: grid;grid-template-columns: repeat(10, 1fr);
gap: 18px; font-size: 0; list-style: none; margin-bottom: 30px;
overflow-x: auto; scrollbar-width: none;
}
.slider-wrapper .image-list::-webkit-scrollbar {
display: none;
}
.slider-wrapper .image-list .image-item {
width: 325px;height: 400px; object-fit: cover;
}
.container .slider-scrollbar {
height: 24px; width: 100%; display: flex; align-items: center;
}
.slider-scrollbar .scrollbar-track {
background: #ccc; width: 100%; height: 4px; display: flex;
align-items: center; border-radius: 4px; position: relative;
}
.slider-scrollbar:hover .scrollbar-track {
height: 4px;
}
.slider-scrollbar .scrollbar-thumb {
position: absolute; background: #000; top: 0;
bottom: 0; width: 50%; height: 100%; cursor: grab; border-radius: inherit;
}
.slider-scrollbar .scrollbar-thumb:active {
cursor: grabbing; height: 8px; top: -2px;
}
.slider-scrollbar .scrollbar-thumb::after {
content: ""; position: absolute; left: 0;
right: 0; top: -10px; bottom: -10px;
}
/* Styles for mobile and tablets */
@media only screen and (max-width: 1023px) {
.slider-wrapper .slide-button {
display: none !important;
}
.slider-wrapper .image-list {
gap: 10px; margin-bottom: 15px; scroll-snap-type: x mandatory;
}
.slider-wrapper .image-list .image-item {
width: 280px; height: 380px;
}
.slider-scrollbar .scrollbar-thumb {
width: 20%;
}
}
Finally, as per expectation we have to add the following JavaScript code to your file script.js file for necessary actions have to be take place like horizontal sliding between images, upon click on any single vector : movement of images front and previous etc., For which we have to use some methods like getBoundingClientRect(), handleMouseMove(), addEventListner(), removeEventListener(), etc.,
const initSlider = () => {
const imageList = document.querySelector(".slider-wrapper .image-list");
const slideButtons = document.querySelectorAll(".slider-wrapper .slide-button");
const sliderScrollbar = document.querySelector(".container .slider-scrollbar");
const scrollbarThumb = sliderScrollbar.querySelector(".scrollbar-thumb");
const maxScrollLeft = imageList.scrollWidth - imageList.clientWidth;
// thumb drag Handle Scrollbar
scrollbarThumb.addEventListener("mousedown", (e) => {
const startX = e.clientX;
const thumbPosition = scrollbarThumb.offsetLeft;
const maxThumbPosition = sliderScrollbar.getBoundingClientRect().width - scrollbarThumb.offsetWidth;
// position on mouse move Update thumb
const handleMouseMove = (e) => {
const deltaX = e.clientX - startX;
const newThumbPosition = thumbPosition + deltaX;
// Ensure the scrollbar when thumb stays within bounds
const boundedPosition = Math.max(0, Math.min(maxThumbPosition, newThumbPosition));
const scrollPosition = (boundedPosition / maxThumbPosition) * maxScrollLeft;
scrollbarThumb.style.left = `${boundedPosition}px`;
imageList.scrollLeft = scrollPosition;
}
// to Remove event listeners on mouse up
const handleMouseUp = () => {
document.removeEventListener("mousemove", handleMouseMove);
document.removeEventListener("mouseup", handleMouseUp);
}
// For drag interaction Add event listeners
document.addEventListener("mousemove", handleMouseMove);
document.addEventListener("mouseup", handleMouseUp);
});
// Slide images according to the slide button clicks
slideButtons.forEach(button => {
button.addEventListener("click", () => {
const direction = button.id === "prev-slide" ? -1 : 1;
const scrollAmount = imageList.clientWidth * direction;
imageList.scrollBy({ left: scrollAmount, behavior: "smooth" });
});
});
// Show or hide slide buttons based on scroll position
const handleSlideButtons = () => {
slideButtons[0].style.display = imageList.scrollLeft <= 0 ? "none" : "flex";
slideButtons[1].style.display = imageList.scrollLeft >= maxScrollLeft ? "none" : "flex";
}
// Update scrollbar thumb position based on image scroll
const updateScrollThumbPosition = () => {
const scrollPosition = imageList.scrollLeft;
const thumbPosition = (scrollPosition / maxScrollLeft) * (sliderScrollbar.clientWidth - scrollbarThumb.offsetWidth);
scrollbarThumb.style.left = `${thumbPosition}px`;
}
// when image list scrolls then Call these two functions
imageList.addEventListener("scroll", () => {
updateScrollThumbPosition();
handleSlideButtons();
});
}
window.addEventListener("resize", initSlider);
window.addEventListener("load", initSlider);
Pingback: Image slider with animation – Coding Jasim