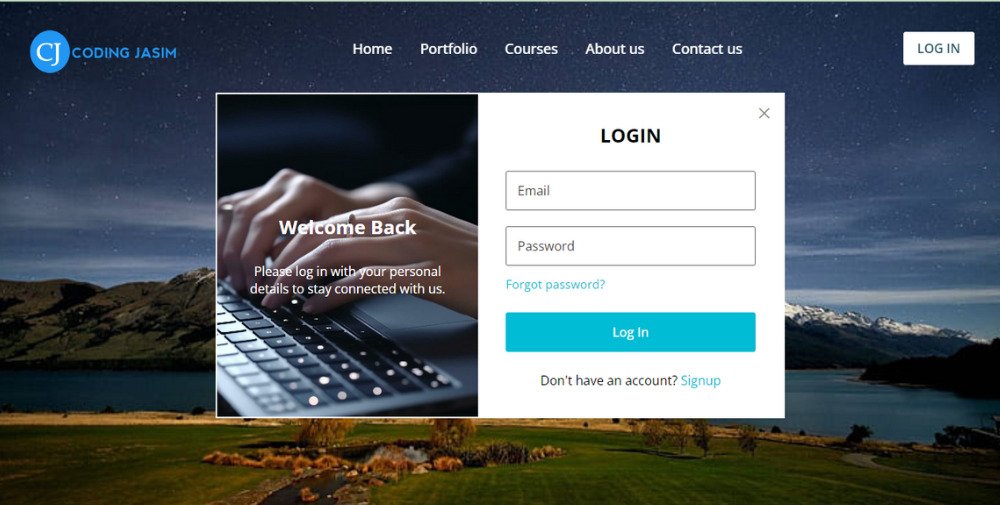
For every new web developers, they should know about these most commonly used basic elements in every webpage development survey :
- Login is a process where users provide credentials, like user name and password, to access secure system or platform. Which will ensures the security by verifying the identity of users before granting them access to restricted areas or personalized content.
- Signup, also known as registration, is the familiar process of creating a new account on a website or application. Which allows users to create personalized account, enabling access to additional features, personalized content, and account management options. Where users typically provide information such as username, password, and email address, etc. After submitting the information, the system creates a new account for the user.
- Navigation menu bar, often referred to as a navbar, is graphical user interface element used to navigate a website or application. It provides users with easy access to different section or pages of the website. Which usually contains links or buttons to essential pages such as Home, About Us, Services, Contact, etc. It may also include dropdown menus or search functionality. The design of the navigation menu bars are typically located at the top of a webpage.
Now with this blog post, every users can easily understand the process of creating a responsive website with login and registration forms using HTML, CSS, and JavaScript. By completing this, you’ll gain practical cum theoretical experience and learn essential web development concepts.
In this website homepage project, contains a navigation menu bar and a login button. When we click on the LOGIN button, a login form will popup with a cool blurred background. The form has an image on left and input field on right side. If you want to Signup instead, simply click on Signup link and it’ll be take to registration form.
The navigation and forms are completely responsive, it means that webpage content will adjust to fit on every screen size. On a smaller screen, the navigation bar will popup from the right side when the hamburger is clicked meanwhile in the forms the left image section will remain hidden.
Video Tutorial of Responsive Website with Navigation bar and Login / Registration form
If you prefer learning through video tutorials, the YouTube video mentioned above is a fantastic resource. Through this video, we have detailed each line of code and provided helpful comments to ensure that the process of building your own website with login and registration forms is accessible and straightforward for beginners.
Nonetheless, if you prefer reading blog articles or wish for a comprehensive tutorial for this project, feel free to continue with this post. By the conclusion of this article, you will have your own website featuring forms that are easy to modify and integrate into your other projects.
Source Code
To build a responsive website with login and registration forms using HTML, CSS, and JavaScript, simply follow these straightforward instructions step by step.
- Let’s create a folder with any specific name as you like. Then, make these below below files inside it.
- Create a structural file called index.html which serve as main file of this project.
- To design this html, then create a file called style.css to hold the CSS code.
- Now for interactive actions to be taken on this html, then create a file called script.js which hold the JavaScript code.
- Finally, download the images folder and place inside it in your project folder.
Now, add the following HTML codes to your index.html file. These codes contains all essential HTML elements, such as header, nav, ul, form and more.
<!DOCTYPE html>
<!-- Coding By Coding Jasim - www.codingjasimweb.com -->
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>A Website with Login & Signup Form | CodingJasim</title>
<!-- Google Fonts Link For Icons -->
<link rel="stylesheet" href="https://fonts.googleapis.com/css2?family=Material+Symbols+Rounded:opsz,wght,FILL,GRAD@48,400,0,0">
<link rel="stylesheet" href="style.css">
<script src="script.js" defer></script>
</head>
<body>
<!--Navigation bar-->
<header>
<nav class="navbar">
<span class="menu-btn material-symbols-rounded">menu</span>
<a href="#" class="logo">
<img src="images/logo.png" alt="logo">
</a>
<ul class="links">
<span class="close-btn material-symbols-rounded">close</span>
<li><a href="#">Home</a></li>
<li><a href="#">Porfolio</a></li>
<li><a href="#">Courses</a></li>
<li><a href="#">About us</a></li>
<li><a href="#">Contact us</a></li>
</ul>
<button class="login-btn">LOG IN</button>
</nav>
</header>
<!--Popup form-->
<div class="blur-bg-overlay"></div>
<div class="form-popup">
<span class="close-btn material-symbols-rounded">close</span>
<div class="form-box login">
<div class="form-details">
<h2>Wecome Back</h2>
<p>Please log in using your personal details to stay connected with us.</p>
</div>
<div class="form-coentnt">
<h2>LOGIN</h2>
<form action="#">
<div class="input-field">
<input type="text" required>
<label>Email</label>
</div>
<div class="input-field">
<input type="password" required>
<label>Password</label>
</div>
<a href="#" class="forgot-pass">Forgot passowrd?</a>
<button type="submit">Log In</button>
</form>
<div class="bottom-link">
Don't have an account?
<a href="#" id="signup-link">Signup</a>
</div>
</div>
</div>
<div class="form-box signup">
<div class="form-details">
<h2>Create Account</h2>
<p>To become a part of our community, please sign up using your personal details.</p>
</div>
<div class="form-coentnt">
<h2>SIGNUP</h2>
<form action="#">
<div class="input-field">
<input type="text" required>
<label>Enter your email</label>
</div>
<div class="input-field">
<input type="password" required>
<label>Create password</label>
</div>
<div class="policy-text">
<input type="checkbox" id="policy">
<label for="policy">
I agree the
<a href="#">Terms & Conditions</a>
</label>
</div>
<button type="submit">Sign Up</button>
</form>
<div class="bottom-link">
Already have an account?
<a href="#" id="login-link">Login</a>
</div>
</div>
</div>
</div>
</body>
</html>
Further, add the following CSS codes to your style.css file to decorate your website and forms. You can also experiment with different CSS properties like colors, fonts, and backgrounds etc., to achieve visual presentation.
/* Importing Google font - Open Sans */
@import url("https://fonts.googleapis.com/css2?family=Open+Sans:wght@400;500;600;700&display=swap");
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: "Open Sans", sans-serif;
}
body{
width: 100%;
height: 100vh;
background: url("images/hero-bg.jpg") center / cover no-repeat;
}
header {
position: fixed;
width: 100%;
left: 0;
top: 0;
padding: 0 10px;
}
.navbar{
display: flex;
padding: 21px 0;
align-items: center;
max-width: 1200px;
margin: 0 auto;
justify-content: space-between;
}
.navbar .menu-btn {
display: none;
color: #fff;
cursor: pointer;
font-size: 1.5rem;
}
.navbar .logo img{
width: 150px;
}
.navbar .links {
display: flex;
list-style: none;
gap: 33px;
}
.navbar .links .close-btn {
display: none;
color: #000;
cursor: pointer;
position: absolute;
right: 20px;
top: 20px;
font-size: 1.5rem;
}
.navbar .links a {
color: #fff;
text-decoration: none;
font-size: 1.1rem;
font-weight: 500;
}
.navbar .links a:hover {
color: #19e8ff;
}
.navbar .login-btn {
border: none;
outline: none;
color: #275360;
font-size: 1rem;
font-weight: 600;
padding: 10px 17px;
border-radius: 2px;
cursor: pointer;
background: #fff;
}
.blur-bg-overlay {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
opacity: 0;
pointer-events: none;
backdrop-filter: blur(5px);
transition: 0.1s ease;
}
.show-popup .blur-bg-overlay {
opacity: 1;
pointer-events: auto;
}
.form-popup {
position: fixed;
top: 50%;
left: 50%;
background: #fff;
max-width: 720px;
opacity: 0;
pointer-events: none;
width: 100%;
border: 2px solid #fff;
transform: translate(-50%, -100%);
}
.show-popup .form-popup {
opacity: 1;
pointer-events: auto;
transform: translate(-50%, -50%);
transition: transform 0.3s ease, opacity 0.1s;
}
.form-popup .close-btn{
position: absolute;
top: 12px;
right: 12px;
color: #878784;
cursor: pointer;
}
.form-popup .form-box {
display: flex;
}
.form-box .form-details {
max-width: 330px;
width: 100%;
color: #fff;
display: flex;
padding: 0 20px;
align-items: center;
flex-direction: column;
text-align: center;
justify-content: center;
}
.login .form-details {
background: url("images/login-img.jpg");
background-size: cover;
background-position: center;
}
.signup .form-details {
background: url("images/signin-img.jpg");
background-size: cover;
background-position: center;
}
.form-box .h2 {
text-align: center;
margin-bottom: 30px;
}
.form-box .form-coentnt {
width: 100%;
padding: 35px;
}
form .input-field {
height: 50px;
width: 100%;
margin-top: 20px;
position: relative;
}
form .input-field input {
width: 100%;
height: 100%;
outline: none;
padding: 0 15px;
font-size: 0.95rem;
border-radius: 3px;
border: 1px solid #717171;
}
form .input-field label {
position: absolute;
top: 50%;
left: 15px;
color: #717171;
pointer-events: none;
transform: translateY(-50%);
transition: 0.2s ease;
}
.input-field input:focus{
border-color: #00bcd4;
}
.input-field input:is(:focus, :valid) {
padding: 16px 15px 0;
}
.input-field input:is(:focus, :valid) ~ label {
color: #00bcdd;
font-size: 0.75rem;
transform: translateY(-120%);
}
.form-box a {
color: #00bcd4;
text-decoration: none;
}
.form-box a:hover {
text-decoration: underline;
}
.form-box :where(.forgot-pass, .policy-text) {
display: inline;
margin-top: 14px;
font-size: 0.9rem;
}
form button {
width: 100%;
outline: none;
border: none;
font-size: 1rem;
font-weight: 500;
padding: 14px;
border-radius: 3px;
margin: 25px 0;
color: #fff;
cursor: pointer;
background: #00bcd4;
transition: 0.2s ease;
}
form button:hover {
background: #0097a7;
}
.form-box .bottom-link {
text-align: center;
}
.form-popup .signup,
.form-popup.show-signup .login {
display: none;
}
.form-popup.show-signup .signup {
display: flex;
}
.signup .policy-text {
display: flex;
align-items: center;
}
.signup .policy-text input{
width: 14px;
height: 14px;
margin-right: 7px;
}
@media (max-width: 950px) {
.navbar :is(.menu-btn, .links .close-btn) {
display: block;
}
.navbar {
padding: 15px 0;
}
.navbar .logo img {
display: none;
}
.navbar .links {
position: fixed;
left: -100%;
top: 0;
width: 100%;
height: 100vh;
display: block;
padding-top: 60px;
text-align: center;
background: #fff;
transition: 0.2s ease;
}
.navbar .links.show-menu {
left: 0;
}
.navbar .links a {
color: #000;
display: inline-flex;
margin: 20px 0;
font-size: 1.25rem;
}
.navbar .login-btn {
font-size: 0.9rem;
padding: 7px 10px;
}
}
@media (max-width: 760px) {
.form-popup {
width: 95%;
}
.form-box .form-details {
display: none;
}
.form-box .form-coentnt {
padding: 30px 20px;
}
}
After applying styles, load the webpage in your browser to view your website, it seems awesome with navigation bar and login button but the forms are currently hidden which can only appear later using JavaScript.
Let’s add the following JavaScript code to your script.js file. The code contains click event listeners which can toggle classes on various HTML elements. If you watch the above video tutorial till end then easily understand all click events which are use in JavaScript code.
const navbarMenu = document.querySelector(".navbar .links");
const menuBtn = document.querySelector(".menu-btn");
const hideMenuBtn = navbarMenu.querySelector(".close-btn");
const showPopupBtn = document.querySelector(".login-btn");
const formPopup = document.querySelector(".form-popup");
const hidePopupBtn = document.querySelector(".form-popup .close-btn");
const loginSignupLink = document.querySelectorAll(".form-box .bottom-link a");
// show mobile menu
menuBtn.addEventListener("click", () =>{
navbarMenu.classList.toggle("show-menu");
});
// hide mobile menu
hideMenuBtn.addEventListener("click", () => menuBtn.click());
// show form popup
showPopupBtn.addEventListener("click", () => {
document.body.classList.toggle("show-popup");
});
// hide from popup
hidePopupBtn.addEventListener("click", () => showPopupBtn.click());
loginSignupLink.forEach(link => {
link.addEventListener("click", (f) => {
f.preventDefault();
formPopup.classList[link.id === "signup-link" ? 'add' : 'remove']("show-signup");
});
});
In Conclusion, through this blog post you can learn about basic concepts related to Login, Sign-up, and Navigation bar. Then further a complete video tutorial of creating basic responsive homepage page by using HTML, CSS & JavaScript followed by codes to improve your web development skills and also experiment with your own from these codes.
If you face any issues while building your website with forms, you are able to download the project’s source cod files for free by clicking Download button.